Difficulty Level = 5 [What’s this?]
UPDATE: I have re-done this project using simple 434MHz RF transmitter/receiver devices. Check out the new project!.
UPDATE: Also see this project for an easy way to display a temperature reading: Digit Shield Temperature Display.
I decided to explore the more advanced features of XBee radios by building a remote temperature sensor. You can get quite a bit of control over an XBee radio without a microcontroller at all. You can configure the radio to send sensor readings at particular intervals when it detects changes on certain input pins. For the details on configuring XBee radios, see the documentation at Digi International.
For this project, I configured the radio at the sensor end to read the analog input of pin 19 every 4 seconds and to send a sensor reading packet. Both the sender and receiver radios must be running the API firmware. This does not work if they are running the default AT firmware. And the “API” parameter is not the same as running the API firmware. You literally need to write a different firmware image to your radios using the X-CTU tool from Digi. I have Series 2 radios, so if you are using Series 1, you need to read the correct documentation for your radios and modify the code.
Input pin 19 on my sensor radio is configured (parameter D1) with value ‘2’ which means that it will read analog input, and the IO sampling rate (parameter IR) is set to ‘1000’ which sends a sample every 4096ms.
An LM34 temperature sensor outputs a variable voltage depending on the temperature. The mapping is extremely simple: 10mV for every Fahrenheit degree. So, at 72 degrees F, the output is 720mV.
Why did I choose pin 19? I started with pin 20, but I burned it out. The pins can only handle an analog input of up to 1.2V, and I think I may have sent too much into the pin. How? Well, let’s say it involved holding a cold Pepsi can on the circuit to cool off the temperature sensor, and I shorted out a connection with the can. Oops. I’m lucky I didn’t burn out the entire XBee chip.
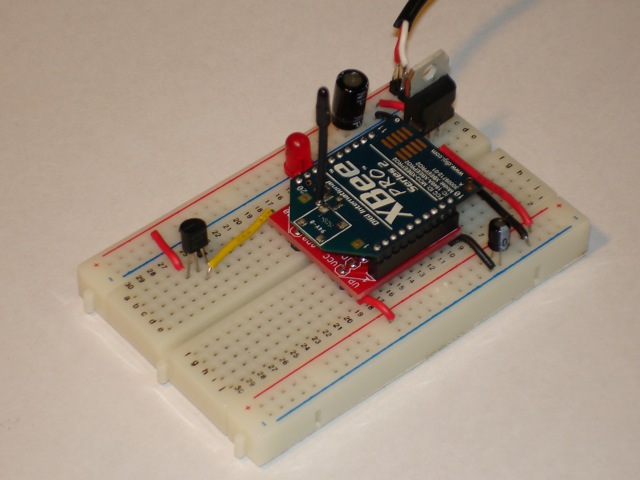
Remote temperature sensor
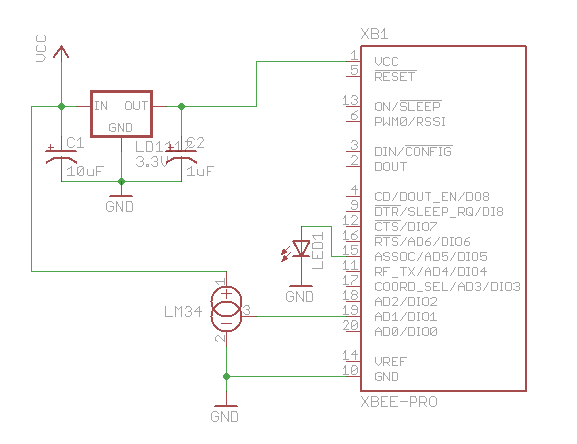
Schematic for remote sensor circuit
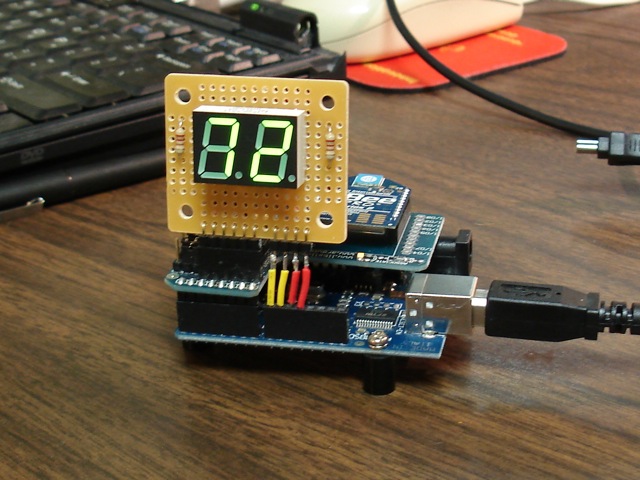
Arduino with XBee shield and LED display
#define NUM_DIGITAL_SAMPLES 12 #define NUM_ANALOG_SAMPLES 4 int groundPins[7] = {8, 2, 3, 4, 5, 9, 7}; int digitPins[2] = {11, 10}; int ON = HIGH; int OFF = LOW; int number[10][7]; int digit[2]; int TOP = 0; int UPPER_L = 1; int LOWER_L = 2; int BOTTOM = 3; int LOWER_R = 4; int UPPER_R = 5; int MIDDLE = 6; int index; int n = 0; int packet[32]; int digitalSamples[NUM_DIGITAL_SAMPLES]; int analogSamples[NUM_ANALOG_SAMPLES]; void setup() { Serial.begin(9600); for(int i=0;i<7;i++) { pinMode(groundPins[i], OUTPUT); } for(int i=0;i<2;i++) { pinMode(digitPins[i], OUTPUT); } initNumber(); setDigit(n); } void loop() { readPacket(); drawDisplay(); } void readPacket() { if (Serial.available() > 0) { int b = Serial.read(); if (b == 0x7E) { packet[0] = b; packet[1] = readByte(); packet[2] = readByte(); int dataLength = (packet[1] << 8) | packet[2]; for(int i=1;i<=dataLength;i++) { packet[2+i] = readByte(); } int apiID = packet[3]; packet[3+dataLength] = readByte(); // checksum printPacket(dataLength+4); if (apiID == 0x92) { int analogSampleIndex = 19; int digitalChannelMask = (packet[16] << 8) | packet[17]; if (digitalChannelMask > 0) { int d = (packet[19] << 8) | packet[20]; for(int i=0;i < NUM_DIGITAL_SAMPLES;i++) { digitalSamples[i] = ((d >> i) & 1); } analogSampleIndex = 21; } int analogChannelMask = packet[18]; for(int i=0;i<4;i++) { if ((analogChannelMask >> i) & 1) { analogSamples[i] = (packet[analogSampleIndex] << 8) | packet[analogSampleIndex+1]; analogSampleIndex += 2; } else { analogSamples[i] = -1; } } } } int reading = analogSamples[1]; // pin 19 // convert reading to millivolts float v = ((float)reading/(float)0x3FF)*1200.0; // convert to Fahrenheit. 10mv per Fahrenheit degree float f = v / 10.0; // round to nearest int n = (int)(f+0.5); setDigit(n); } } void drawDisplay() { for(int g=0;g<7;g++) { digitalWrite(groundPins[g], LOW); for(int i=0;i<2;i++) { if (digit[i] < 0) { continue; } digitalWrite(digitPins[i], number[digit[i]][g]); } delay(0); // for some reason, this is required even if the value is 0 digitalWrite(groundPins[g], HIGH); } } void setDigit(int n) { n = n % 100; digit[0] = n % 10; digit[1] = (n / 10) % 10; if ((digit[1] == 0) && (n < 10)) { digit[1] = -1; } } void initNumber() { number[0][0] = ON; number[0][1] = ON; number[0][2] = ON; number[0][3] = ON; number[0][4] = ON; number[0][5] = ON; number[0][6] = OFF; number[1][0] = OFF; number[1][1] = OFF; number[1][2] = OFF; number[1][3] = OFF; number[1][4] = ON; number[1][5] = ON; number[1][6] = OFF; number[2][0] = ON; number[2][1] = OFF; number[2][2] = ON; number[2][3] = ON; number[2][4] = OFF; number[2][5] = ON; number[2][6] = ON; number[3][0] = ON; number[3][1] = OFF; number[3][2] = OFF; number[3][3] = ON; number[3][4] = ON; number[3][5] = ON; number[3][6] = ON; number[4][0] = OFF; number[4][1] = ON; number[4][2] = OFF; number[4][3] = OFF; number[4][4] = ON; number[4][5] = ON; number[4][6] = ON; number[5][0] = ON; number[5][1] = ON; number[5][2] = OFF; number[5][3] = ON; number[5][4] = ON; number[5][5] = OFF; number[5][6] = ON; number[6][0] = ON; number[6][1] = ON; number[6][2] = ON; number[6][3] = ON; number[6][4] = ON; number[6][5] = OFF; number[6][6] = ON; number[7][0] = ON; number[7][1] = OFF; number[7][2] = OFF; number[7][3] = OFF; number[7][4] = ON; number[7][5] = ON; number[7][6] = OFF; number[8][0] = ON; number[8][1] = ON; number[8][2] = ON; number[8][3] = ON; number[8][4] = ON; number[8][5] = ON; number[8][6] = ON; number[9][0] = ON; number[9][1] = ON; number[9][2] = OFF; number[9][3] = ON; number[9][4] = ON; number[9][5] = ON; number[9][6] = ON; } void printPacket(int l) { for(int i=0;i < l;i++) { if (packet[i] < 0xF) { // print leading zero for single digit values Serial.print(0); } Serial.print(packet[i], HEX); Serial.print(" "); } Serial.println(""); } int readByte() { while (true) { if (Serial.available() > 0) { return Serial.read(); } } }
Here’s how I wired the back of the LED display. The code displays each digit separately to save Arduino pins but toggles between the digits so fast you can’t see any flicker.
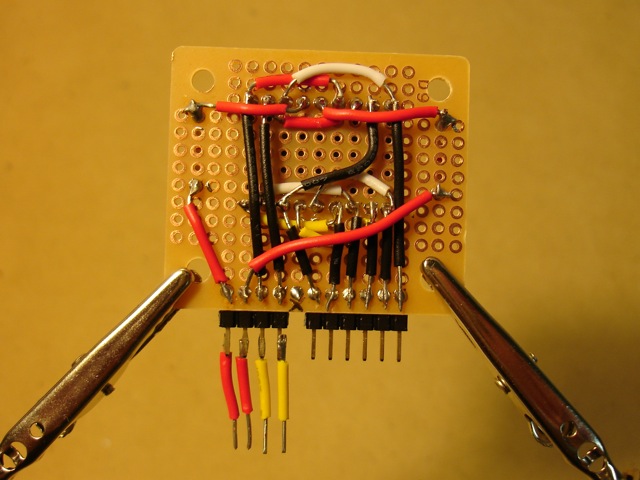
Back of LED display. The pin positions are designed to fit the XBee shield.
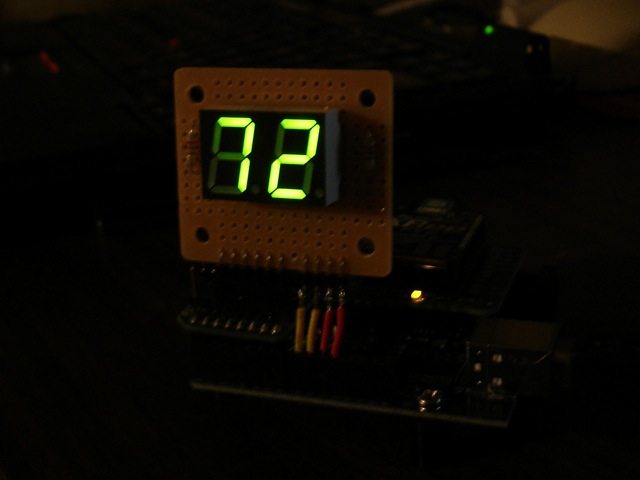
In the dark!
// convert reading to millivolts
float v = ((float)reading/(float)0x3FF)*1200.0;
// convert to Fahrenheit. 10mv per Fahrenheit degree
float f = v / 10.0;
// round to nearest int
n = (int)(f+0.5);
change the calculation at here?
@billy: the LM35 reports 10mv per celsius degree. If you want to display Fahrenheit, convert celsius to Fahrenheit in your code.
float c = (5.0/9.0)*(f-32.0)
Massive progress !!! A mate suggested I use the XB-24ZB firmware (basically a ZigBee upgrade for my XBee 2.5) and I then got the IO Sampling menu. I have set it all up and now I get, every 5secs, a temp value at the Arduino !!!!
Temp: 0
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 01 02 88 87
Temp: 0
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 01 02 88 87
Temp: 0
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 01 02 88 87
Temp: 0
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 01 02 88 87
Temp: 0
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 01 02 88 87
Temp: 0
Problem is. I am now getting the Temp: 0 thing.
I am one step closer though !! Woo Hoo !
@ilium007: your packets look good, but it looks like you have the sensor connected to pin 20 on the XBee? The code looks for the sample on pin 19. The temperature is in these two bytes near the end of the packet: 02 88
To get temperature, this value is divided by the max value 0x3FF and multiplied by 1200.
So, (0x288/0x3FF) * 1200 = 760.1173mv
At 10mv per degree, your temperature reading is 76 degrees.
If you are using pin 20, change this line:
int reading = analogSamples[1]; // pin 19
to this:
int reading = analogSamples[0]; // pin 20
i facing another big problem! how could i know my xbee can communicate each other. i using X-CTU to change their address already by follow the instruction from datasheet.besides, after i upload the programming code to my arduino board,then i connect to X-CTU.when i click TEST.It show UNALBE TO COMMUNICATE.
@Michael – this is really helpful, thanks. I am still at a bit of a loss to the 1200. My TMP36 (centigrade) sensor does not seem to have an upper mv rating, in the data sheet I see 1000mv at 50 degrees centigrade in the table but I can’t find an upper limit. Do you just work out the range at which your are measuring and divide the 1024 digital resolution across that ? So say that I wanted to measure up to 50 degrees centigrade, I would take the ADC output / 1023 (why that is not 1024 I don’t know) and then multiply by 1000 (1000mv at 50 degrees). Would this then give me a mV output in the range of 0 to 50 degrees centigrade?
If you have a moment and could take a look at the data sheet that would be awesome. http://www.analog.com/static/imported-files/data_sheets/TMP35_36_37.pdf on page 8.
@ilium007:
The sensor outputs 10mv per degree, no matter what it is connected to, and no matter what range you want to measure.
1200mv is a characteristic of the XBee pin, not the sensor. The analog input pin on the XBee can measure 0-1200mv. It reports this voltage measurement as a value from 0-1023. So if the XBee ADC reports a value of 300, then this is (300/1023) * 1200 = 351.9mv. 351.9mv is 35.19 degrees.
The value is 1023 (not 1024) because 1023 is the largest number you can represent with 10 bits. It is a 10 bit ADC. It can represent 1024 values, and the values are 0-1023. The zero counts.
I think I have an answer – will try this tonight:
(from http://www.ladyada.net/learn/sensors/tmp36.html)
Voltage at pin in milliVolts = (reading from ADC) * (5000/1024)
This formula converts the number 0-1023 from the ADC into 0-5000mV (= 5V)
If you’re using a 3.3V Arduino, you’ll want to use this:
Voltage at pin in milliVolts = (reading from ADC) * (3300/1024)
This formula converts the number 0-1023 from the ADC into 0-3300mV (= 3.3V)
Then, to convert millivolts into temperature, use this formula:
Centigrade temperature = [(analog voltage in mV) – 500] / 10
@ilium007: is your sensor connected to the Arduino or the XBee? I thought you were reading a temperature at the XBee and transmitting the value to the Arduino? If so, the ADC on the Arduino has nothing to do with this! You are not measuring any voltages at the Arduino. You are simply receiving serial data from an XBee that measured a voltage with its ADC.
Yeah I get it. I am not measuring anything on the Arduino (the little code sample I included was for reading direct from an Arduino but I am not doing this – I included just for the purposes of the calculation). I am simply using the Arduino to run the code that reads the Din and Dout from the receiving XBee. I was not aware the ADC pin on the XBee only read up to 1200mv (reading XBee doco again now ;) ) – so what happens when the TMP36 delivers a max of 5v out its signal pin ? With the 500mV offset at zero on that sensor, at 25 degrees centigrade I should see 750mV on the centre pin of the TMP36 and at 50 degrees centigrade I would see 1500mV which would do what to the ADC input on the XBee ? Excuse me if I sound ignorant, but even if 1023 is the largest number that can be represented with a 10 bit number, there are still 1024 steps between 0 and 1023 – is there not ?
In your calculation above, the 300 (ADC) relating to 35.19 degrees, last night in my air conditioned office (probably around 21 degrees centigrade) the ADC pin was reporting around 623. Something is way off there. I might go and buy another TMP36 and see if I get a different reading.
From page 96 of the XBee Guide ( http://ftp1.digi.com/support/documentation/90000976_G.pdf ):
Analog samples are returned as 10-bit values. The analog reading is scaled such that 0x0000 represents 0V, and
0x3FF = 1.2V. (The analog inputs on the module cannot read more than 1.2V.) Analog samples are returned in order
starting with AIN0 and finishing with AIN3, and the supply voltage. Only enabled analog input channels return data
as shown in the figure below.
To convert the A/D reading to mV, do the following:
AD(mV) = (A/D reading * 1200mV) / 1024
They are dividing by 1024 to get a mV reading – is this correct ?
You’re right that the sensor will not work with the XBee ADC if it outputs a value greater than 1.2V. You will burn out the input pin on the XBee (I think). I didn’t know your sensor had an offset of 500mV at 0 degrees. That gives you a bit of a problem and you won’t be able to measure temperatures as high as you want.
Yes, 1023 is the maximum value, but there are 1024 possible values because you count zero as a value. If you test the formula at the upper limit, it becomes more clear.
Regarding the formula from the datasheet — that formula looks incorrect. They should divide by 1023.
Look at it this way: It says that the value 0x3FF (which is 1023) represents 1.2V (1200mV). So plug this highest possible reading into the formula and see if it works: (1023 * 1200) / 1024. Does this give 1200mV? Nope. But (1023 * 1200)/1023 certainly is 1200mV. You have to divide by the maximum value, not the number of possible values. I have to think about this every time, too, and then I just test at the maximum to make it more clear in my mind.
Cool – I will give it another go tonight. Thanks for your efforts.
@Michael – just looking at the sensor you are using, I see this:
Supply Voltage +35V to −0.2V
Output Voltage +6V to −1.0V
Output Current 10 mA
So your sensor could theoretically go higher than 1200mV ? What should I be looking for in a sensor ? It seems most temp sensors I have found go well above 1200mV.
But on the other hand, even with my 500mV offset at 0 degrees C, at 25 degrees C I should see 750mV, at 50 degrees C I should see 1000mV and I could go up to 70 degrees for the ADC ceiling of 1200mV.
I hope this is correct.
Yes, that’s true, my sensor can output more than 1200mV. But I simply didn’t put it in an environment where that would happen. Sounds like you can go up to 70 C “safely”.
Hot Dog we have a Weiner…..
Temp: 28
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 02 02 92 7C
Temp: 27
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 02 02 92 7C
Temp: 27
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 02 02 91 7D
Temp: 27
7E 00 12 92 00 13 A2 00 40 64 F7 5D 1B 91 01 01 00 00 02 02 90 7E
Temp: 27
@Michael – thanks for all your help mate.
int reading = analogSamples[1]; // pin 19
// convert reading to millivolts
float v = ((float)reading/(float)0x3FF)*1200.0;
// convert to Fahrenheit. 10mv per Fahrenheit degree
float f=v/10.0;
float c =(5.0/9.0)*(f-32.0);
@Michael-after i put this i still cant communicate with my remote temperature sensor board? is that any problem to fix it?im worrying about the communication of my xbee on remote temperature sensor board and xbee shield.beside X-CTU i still can use what software to check their communication? my 7 segment show double 00.it cant sense any temperature. can teach me how to get it right? thanks.
@billy, use the print statements in the code to output the packets (like ilium007 did above). Also, isn’t your sensor a Celsius censor and you want to convert to Fahrenheit? I have to the formula for converting F to C. You need to convert from C to F, so solve for f in the equation.
f = (9.0/5.0)*c+32
@Michael, i facing a problem..when upload the code into arduino board. It unable to communicate with my laptop. But i put in other code .like LED blinking code.it can communicate with my laptop.so the print statement is a part from X-CTU?
You understand that X-CTU is only for configuring your modems, right? After configuration, you don’t use X-CTU as part of the project at all.
@Michael- Mean that after i use X-CTU change the address of 2 xbee to let them communicate.i wont use it anymore? how can i show the output like (illium007) can you teach me? for the programming part i just add the formular that u gv me just now f = (9.0/5.0)*c+32; is put in which part of the code? cos after i put i got an error on the f and c.thanks for helping.
@billy, Correct, you don’t use X-CTU after configuring the modems. Slow down and think about what you are trying to accomplish. This project is about a remote sensor attached to an XBee which transmits the sensor readings to an Arduino to display. What role would X-CTU play?
I’ve already documented everything in the project. I’ve explained how to configure the modems, how to wire the sensor, and provided all the code for parsing the packets. You can get it working if you try. Since your sensor will transmit Celsius, you can translate to Fahrenheit if you want, and I gave you the formula. You can get the code working if you TRY. Just slow down and look at the code carefully to figure it out. If you are not able to do C programming, then this is not the right project for you, but if you want to LEARN, then you can try and with some work you will figure it out.
@Michael- i have construct all the circuit and the arduino part and 7segment have already display the double 00.just i cant get the temperature.i have try many time on this project. i follow all the instruction it just the LM 34 i change it to LM 35. can u show me the calculation part with using LM 35 temperature sensor. Im first time handling this arduino. im still learning. Hope you can teach me.thanks.
@billy, I already gave you the formula (which you could have found using Google). If c is the temperature in Celsius, then f = (9.0/5.0)*c+32
LOOK AT YOUR CODE AND TRY AGAIN. And read this: http://nootropicdesign.com/projectlab/difficulty-levels/
I’m having a problem with some LM34’s in a circuit very similar to this one. Both sensors are consistently reading about 5 degrees high when compared to a calibrated thermometer held touching the sensor. Is this something you’re seeing as well?
@Michael or @ilium007 I’m having a very difficult time loading the API firmware onto my XBee and am therefore, currently, only getting 14 bytes in my packet as ilium0007 was. http://www.sparkfun.com/products/8664 This is the XBee that I’m using. I can’t seem to get it to run the API firmware at all. It gives me an error every time. If you could possibly walk me through setting up the configuration of the XBees I would really appreciate it because I have been working on this for ages and finally realized what the problem was only to have it be what seems like impossible to fix. I would really appreciate your help because I think I have modified your code to do exactly what I need however can’t figure out if it works because I’m not getting the proper packet. Thank you soooo much in advance especially since you’ve been so helpful in the past! I hope to hear from you soon!
@EngGirl88:
I’m not sure what problem you are having. You said “I can’t seem to get it to run the API firmware at all. It gives me an error every time.” What do you mean? Do you mean that you are unable to burn the API firmware onto your XBee radios using the X-CTU tool? What errors? Errors from what?
Write the coordinator API firmware on one device, and end device API firmware on the other device. On the sensor radio (the transmitter), set parameter D1 to value 2. And set IR to value 1000. Use X-CTU to do all of this.
If you are having problems, please describe the problems in detail. Tell us exactly what you are doing and exactly what errors you get.
Yes, I meant that I was unable to burn the API firmware onto my XBee radios using the X-CTU tool. I will walk you through the steps that I took attempting to configure the XBee on the sensor end and the errors that I got in each step. Ok… so I opened X-CTU as usual and chose the appropriate serial port and selected Test/Query at which time I got a pop-up which said:
Communication with Modem. OK
Modem Type XB24
Modem Firmware Version 10CD
So that step worked fine. Then I read the parameters which worked fine as well. Then I switched some of the settings under the modem configuration to:
Modem Type: XB24-ZB
Function Set: Zigbee End Device API
I then selected write and this is where things fall apart. I got the following errors:
A pop up: Unable to program module. Incompatible function set selected for current module.
On the bottom “progress” section:
Getting Modem Type… OK
Programming Modem… Incompatible modem selected for programming
Write Parameters… Failed
Sorry this response was so long but I tried to make the response as detailed as possible. I tried so many things I thought I bricked my XBees but was able to “reset” them through a procedure I found online thankfully. But still get the errors above. Thanks so much again for all of your help and I look forward to hearing back from you soon!
Ok, you have a XB24 modem. Now just try to write the API firmware to it. Don’t change settings. Just find the firmware version that is the API firmware for your XB24 modem and write it. THEN you can worry about changing settings in the modem. Also, the vendor has support forums for their product. http://forums.digi.com/support/forum/index
Ok… I am still unsure how to write the API firmware to the modem… I thought that’s what I was attempting to do in the steps I outlined before. The only choice I have that looks remotely like this is to set AP=1 (API Enabled) however I still don’t get the proper sized packets and from what I have read this is not actually API mode. Sorry to bother you but I’m sort of stuck here and it seems as though everywhere I look doesn’t quite have information that is actually helpful (at least not in terminology I understand). Thanks so much again!
Did you read the manual for X-CTU?
If you google “X-CTU manual”, the first hit is the manual. http://ftp1.digi.com/support/documentation/90001003_a.pdf
You have an XB24 modem. You do not have an XB24-ZB modem.
1) Read firmware.
2) Change function set to API firmware. Either Coordinator or end device.
3) Write.
DON’T change the modem from XB24 to XB24-ZB. You said you have an XB24 modem. You can’t write firmware for a different product onto your radio.
Thank you for your timely reply yet again. I am slightly concerned however because I don’t seem to have API firmware in the function set options. The options that I do have are:
XBEE 802.15.4
XBEE 802.15.4 ANALOG IO ADAPTER
XBEE 802.15.4 DIGITAL IO ADAPTER
XBEE 802.15.4 RS232 ADAPTER
XBEE 802.15.4 RS232 POWER HARVESTER
XBEE 802.15.4 RS485 ADAPTER
XBEE 802.15.4 SENSOR ADAPTER
XBEE 802.15.4 USB ADAPTER
Thank you for guiding me to the X-CTU manual since I did not know there was a manual for that however I am still at a loss.
@EngGirl88,
Perhaps your device is not capable of API firmware. I don’t know about every XBee device, just the ones I have. Please consult the Digi documentation and support forums to learn about your device. Digi has a huge support forum so you should ask questions there.
Hi nootropic design,
I am a little simplify your code (writes the temperature into the serial port of the PC).
It works well, but after about one hour, the arduino makes four times restart and then working normaly again. This is repeated.
For more informations: http://arduino.cc/forum/index.php/topic,56276.0.html
Please, could someone help me.
Thanks for reply, George.
Hi Good Tutorial,
I am using a similar method to read an IMU that outputs an analog voltage reading. I am reading two analog inputs (DI00 and DI01). The problem I am having is that the data I receive is not in the format that is specified in the manual or in this tutorial http://www.digi.com/support/kbase/kbaseresultdetl.jsp?kb=180
Here is a sample of the data:
7EFE03FF03FE03FF03FE03FF03FFFE03
7E03FF03FE73FF03FE03FF03FE03FF03
7E001C835678220005060003FF03FE03
7EFE72FF03FE03FF03FF03FF03FE0303
7E001C835678220005060003FF03FE03
7E03FF03FF03FE03FF03FF03FF03FE03
7EFE03FF03FD7503FD03FF03FD03FE03
7E001C8356782200050600FE76FF0303
7E03FE73FF03FE03FF03FD03FF03FD03
7E001C835678220005060003FF03FF03
7E7403FE03FF03FD03FF03FD03FFFF03
7E03FF03FF03FD7103FF03FF03FEFF03
7E001C835678220005060003FD74FF03
7EFF03FE7203FE03FF03FE03FF03FF03
I am using XB24 version 10E8. I have function set as XBEE 802.15.4 and ATAP = 1 (API Enable). The base and the receiver modules are set up basically as they say in the above tutorial. Not sure what I am doing wrong. Any help is appreciated.
Thanks
Did you write the API firmware to the radios?
The API parameter has nothing to do with running the API firmware.
Ok I looked around and found this tutorial http://www.instructables.com/id/Configuring-XBees-for-API-Mode/ for writing API firmware to xbee and it seems that the xbee has to be series 2 ( I think). I will check the ones I have when I get a chance but I was wondering if it is safe to write XB24-B to XB24.
Also I tried just ignoring the messages that do not have (5678) as the source bytes and I seem to be getting the correct messages although I think too few messages are coming too slowly. Is this an ok workaround?
Thanks for your help.
u tutorial is so nice and how u design the Arduino with XBee shield and LED display i cant understand can clearly explain
manikandan, I don’t understand your question.
I am sorry for my ignorance but I am a little unclear as to what the transmitting xbee module is plugged into. Is that a xbee shield of some sort?
Thanks
SteveD, that’s just a breakout board to translate the 2mm pin spacing on the XBee to breadboard-friendly 0.1″ spacing.
Wow, I’ve searched high and low for zigbee temperature sensors and nobody seems to have anything like this! I’m definitely going to build this.. amazing work!
Will this setup work for me to be able to read multiple sensors sprinkled throughout a building? can the receiver end pick which temp sensor to query?
thanks!!!
rob
You can set up a mesh network with multiple sensors sending data to the coordinator. That’s a more sophisticated setup, so you’ll need to read the XBee documentation carefully to understand all that.
I would like to read more than one ADC channel, when I try to measure two channels the results are incorrect. Adjust one ADC level gives a change to the other. Can someone help with me?
Thanks
xsilvergs, did you configure the sending XBee to use two ADC pins? I’ve used two ADC pins on an XBee and not had any interference.
Hi Michael,
I’m trying to implement your project. I’m using xbees (series 1) and a Fio Arduino. Before I add the temperature sensor, I’m trying to connect a direct source (3V to VCC and VREF and 0.101V to pin 19) to see if I can get that value (the equivalent in Fahrenheit). I’m using XCUT to set the xbees.
1) Xbee + Arduino (I set the xbee to “end device”)
2) Xbee + Power Source (I set pin 19 to option 2 (ADC), I also set this one to be the “coordinator” and I set the sample rate to “1000”)
I loaded your code and I got the following error:
7E 00 0A 83 00 00 3C 00 01 04 00 00 00 3B
Temp: 0
7E 00 0A 83 00 00 44 00 01 04 00 00 00 33
Temp: 0
7E 00 0A 83 00 00 3C 00 01 04 00 00 00 3B
Temp: 0
7E 00 0A 83 00 00 3D 00 01 04 00 00 00 3A
Temp: 0
7E 00 0A 83 00 00 45 00 01 04 00 00 00 32
Temp: 0
7E 00 0A 83 00 00 48 00 01 04 00 00 00 2F
Temp: 0
7E 00 0A 83 00 00 47 00 01 04 00 00 00 30
Temp: 0
Is there a way to fix this? Do you think my error has to do with the configuration of the xbees or the code itself? I’ll appreciate your help.
-Sergio
Sergio, my code is for Series 2 XBee radios. Did you write the API firmware to the devices? They need to be running the API firmware.
Michael,
I’m sure the Xbee sends the correct data, I feel the problem is the Arduino code which I don’t understand fully. Why the digitalChannelMask ?
The code received on the coordinator is: 7E 00 14 92 00 13 A2 00 40 6A 4E E6 7D C1 01 01 00 00 06 00 00 03 FF 92
The end device is set to use ADC1 and ADC2 (ADC0 not used)
Your packet looks correct, and the code will properly parse it. The digital channel mask is a two bytes that indicates which pins have digital inputs set. It is packet[16] and packet[17] in your packet, and the values are 00 and 00. This is correct. You didn’t configure your sending XBee for digital inputs.
The analog channel mask indicates which analog pins are set to as inputs. It is packet[18], and the value is 0x06. This means that there are samples for ADC1 and ADC2, which is correct. Each sample is two bytes and they start at packet[19]. So the sample for ADC1 is 0x0000, and the sample for ADC2 is 0x03FF. The last byte of the packet 0x92 is the checksum.
So the packet and code are correct. The values being transmitted are 0x0000 and 0x03FF. 0x03FF is 1023 and is the maximum value, and represents 1200 mV
Are you aware that the ADC channels on the XBee can only handle 1200 mV? If the output range of your sensor is more than 1.2V, then you can damage the XBee.
Michael,
Thank you for what must be the best explanation I’ve read on the net.
I now have now shortened code for the Arduino and have a working example.
Thanks again.
Tony
I’ve just realised that one axis doesn’t change, what have I done wrong?
#define NUM_ANALOG_SAMPLES 4
int packet[32];
int analogSamples[NUM_ANALOG_SAMPLES];
int ADC1, ADC2, ADC3;
void setup()
{
Serial.begin(9600);
}
void loop() {
readPacket();
}
void readPacket() {
if (Serial.available() > 0) {
int b = Serial.read();
if (b == 0x7E) {//find first byte in string
packet[0] = b;
packet[1] = readByte();
packet[2] = readByte();
int dataLength = (packet[1] << 8) | packet[2];
for(int i=1;i<=dataLength;i++) {
packet[2+i] = readByte();
}
int apiID = packet[3];
packet[3+dataLength] = readByte(); // checksum
printPacket(dataLength+4);
if (apiID == 0x92) {
ADC1 = (packet[19] << 8) | packet[20];
ADC2 = (packet[21] << 8) | packet[22];
ADC3 = (packet[23] << 8) | packet[24];
}
}
}
}
void printPacket(int l) {
for(int i=0;i < l;i++) {
if (packet[i] 0) {
return Serial.read();
}
}
}
Any help much appreciated.
iam new in interfacing modules.
i want to plug 3 different sensors with interfacing modules and then transmit the values by modules in a same floor.
pls guide.
hi.
I have a problem with the format of packets.
The xbee is Series2 xb24-zb
Coordinator settings are:
API ENABLE “2” and the IO sampling rate is set to “1000”
End Devices :
API ENABLE “2” , pin AD1 “2” and the IO sampling rate is set to “1000”
The packet that comes out is:
7E 00 12 92 00 7D 33 A2 00 40 33 19 34 FC 9A 41 01 00 00 02 00 A3
It’s wrong!
Any idea??
Hi
What is the software u used to draw the schematic?
Thanks
Schematics drawn with Cadsoft Eagle.
hi
I have my senior project about doing something like this. It is about temperature and humidity but I need to take the data and transmit them to a computer wirelessly.
So please I need a reply on my mail as fast as possible.
Thank you so much.
Firas, I’m not sure what your question is…
i need to get a temperature sensor and humidity sensor, collect data from them,put the data on a buffer,move these data using wireless data transmission to a server,show these data on the laptop
thats what should be done.
Firas, sounds like a good project!
ya so how can i get the help